Styling links within a specific <div>
is a common requirement when designing a web page. You may want to customize link colors, hover effects, or even remove underlines—but only for links inside a particular section of your website. This ensures that other links on the page retain their default or global styling.
In this guide, we will explore different methods to achieve this using CSS. We will also touch upon best practices to keep your styles maintainable and avoid unwanted conflicts.
Using CSS to Target Links in a Specific Div
CSS allows you to style specific elements inside a container by using a class or an ID selector. Here’s how you can do it:
1. Select Links Within a Specific Class
If your container has a class, you can target all the links inside it like this:
/* Style all links inside div with class "custom-links" */
.custom-links a {
color: blue;
text-decoration: none;
font-weight: bold;
}
In this example:
- The text color of links inside the
div
will be blue. - The underline will be removed.
- The text will be bold.
2. Hover Effects for Links in the Div
To enhance user experience, you can add a hover effect specifically for these links:
.custom-links a:hover {
color: red;
text-decoration: underline;
}
Now, when users hover over these links, the color will turn red and an underline will appear.
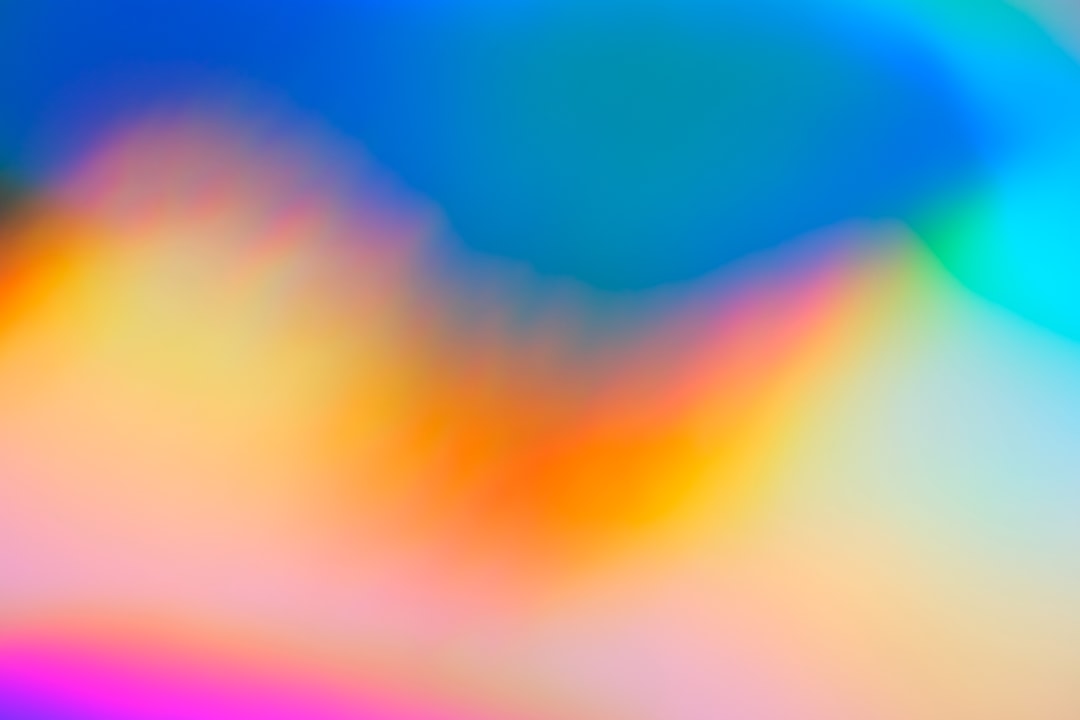
Targeting Links Using an ID Selector
Sometimes, instead of a class, your container might have an ID. If so, you can use an ID selector:
#specific-container a {
color: green;
text-decoration: none;
}
This ensures only links inside the <div id="specific-container">
are affected.
Handling Nested Links
If your <div>
contains other elements that also have links, you may need to be more specific with your selectors. For example:
.custom-links > a {
color: navy;
}
This rule applies only to direct child links inside .custom-links
, and not those inside other elements within it.
Adding a Transition Effect
Smooth transition effects can make hover interactions more appealing. Here’s how to add a fade effect:
.custom-links a {
color: darkblue;
transition: color 0.3s ease-in-out;
}
.custom-links a:hover {
color: orange;
}
Now, when the user hovers over a link, the color transition appears smoothly.
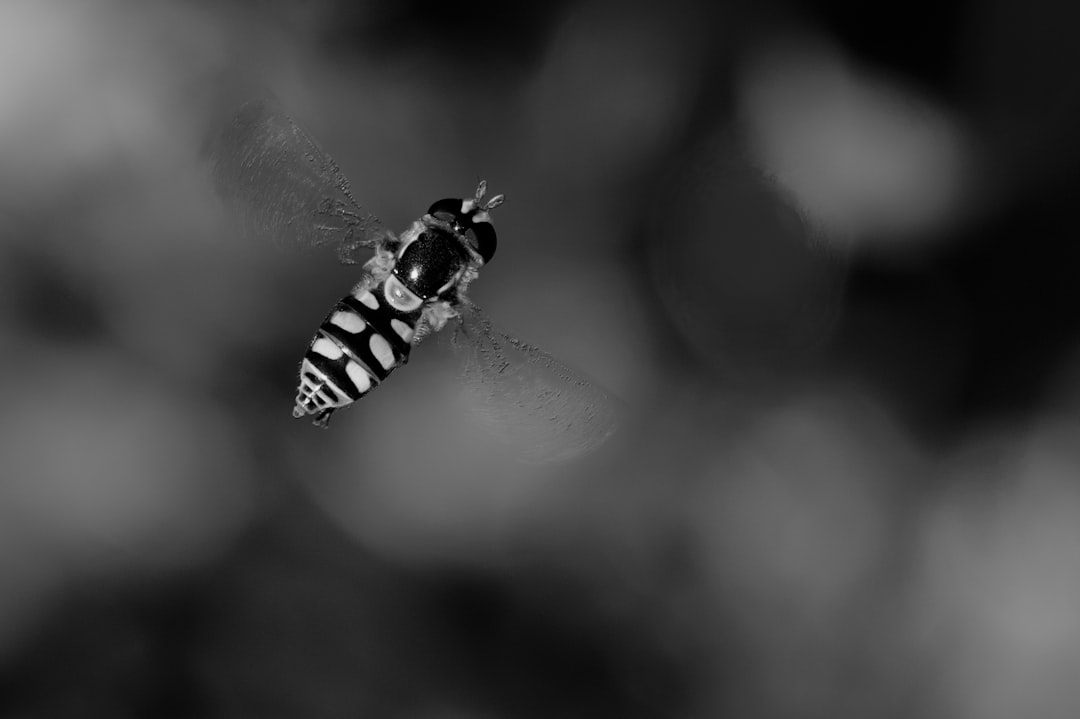
When to Use Inline Styles (And When Not To)
While it’s possible to apply styles inline, doing so is not recommended for maintainability. However, if you must use it for a specific case, you can:
<div class="custom-links">
<a href="#" style="color:purple; text-decoration:underline;">Inline Styled Link</a>
</div>
This approach is generally discouraged because it can be hard to update later.
Ensuring Mobile-Friendliness
Always check your styles on mobile devices. Use media queries to adjust styles when needed:
@media (max-width: 600px) {
.custom-links a {
color: crimson;
font-size: larger;
}
}
With this media query, links inside .custom-links
will change to a crimson color and appear larger on small screens.
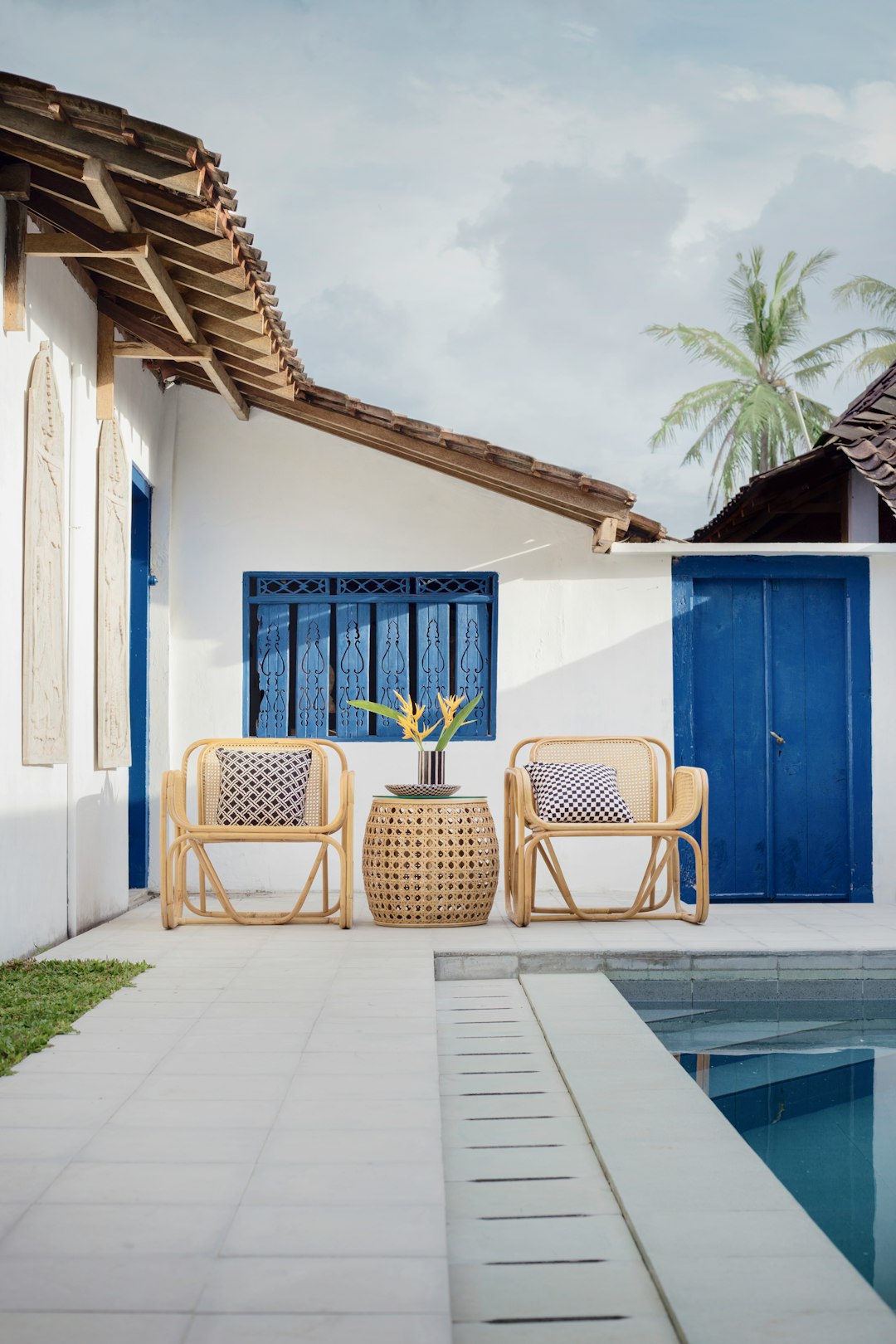
Final Thoughts
By using CSS class or ID selectors, you can selectively style links inside specific <div>
elements without affecting global styles. Adding hover effects, smooth transitions, and considering mobile responsiveness ensures an optimal user experience.
Now that you know how to style links in a specific div, experiment with different colors, underlines, and effects to create a visually appealing design that enhances your website’s usability!
